Custom Shipping Method is a smart move if you want to show shipping methods according to your needs using some parameters/fields in woocommerce.
To create a Custom Shipping Method, You will have to create a plugin using below code.
- Create a folder custom-shipping-method in wp-content/plugins.
- Create a file inside custom-shipping-method folder named custom-shipping-method.php and paste the code below.
<?php
/*
Plugin Name: Custom Shipping Method
Plugin URI: #
description: Custom Shipping Rates and Delivery
Version: 1.0
Author: Sanchit Varshney
Author URI: #
License: GPL2
*/
if ( ! defined( 'ABSPATH' ) ) {
exit; // Exit if accessed directly
}
if (
in_array(
'woocommerce/woocommerce.php',
apply_filters('active_plugins', get_option('active_plugins'))
)
) {
//Add Custom Shipping Method
add_action( 'woocommerce_shipping_init', 'custom_shipping_method' );
function custom_shipping_method() {
if ( ! class_exists( 'Custom_Shipping_Method' ) ) {
class Custom_Shipping_Method extends WC_Shipping_Method {
/**
* Constructor for your shipping class
*
* @access public
* @return void
*/
public function __construct() {
$this->id = 'custom';
$this->method_title = __( 'Custom Shipping', 'custom' );
$this->method_description = __( 'Custom Shipping Method', 'custom' );
$this->init();
$this->enabled = isset( $this->settings['enabled'] ) ? $this->settings['enabled'] : 'yes';
$this->title = isset( $this->settings['title'] ) ? $this->settings['title'] : __( 'Custom Shipping', 'custom' );
$this->availability = 'including';
// Country is set to Romania by Default
$this->countries = array('RO');
}
/**
* Init your settings
*
* @access public
* @return void
*/
function init() {
// Load the settings API
$this->init_form_fields();
$this->init_settings();
// Save settings in admin if you have any defined
add_action( 'woocommerce_update_options_shipping_' . $this->id, array( $this, 'process_admin_options' ) );
}
/**
* Define settings field for this shipping
* @return void
*/
function init_form_fields() {
// We will add our settings here
$this->form_fields = array(
'enabled' => array(
'title' => __( 'Enable', 'shiprocket' ),
'type' => 'checkbox',
'description' => __( 'Enable this shipping.', 'custom' ),
'default' => 'no'
),
'bucharest_fan_courier' => array(
'title' => __( 'Bucharest (Fan Courier)', 'custom' ),
'type' => 'number',
'description' => __( 'Bucharest (Fan Courier) delivery charges.', 'custom' ),
'default' => 14.99
),
'bucharest_beefast' => array(
'title' => __( 'Bucharest (Beefast)', 'custom' ),
'type' => 'number',
'description' => __( 'Bucharest (Beefast) delivery charges.', 'custom' ),
'default' => 9.99
),
'ilfov_fan_courier' => array(
'title' => __( 'Ilfov (Fan Courier)', 'custom' ),
'type' => 'number',
'description' => __( 'Ilfov (Fan Courier) delivery charges.', 'custom' ),
'default' => 14.99
),
'gls' => array(
'title' => __( 'Rest of Country (GLS)', 'custom' ),
'type' => 'number',
'description' => __( 'Rest of Country (GLS) delivery charges.', 'custom' ),
'default' => 16.99
)
);
}
/**
* This function is used to calculate the shipping cost. Within this function, we can check for weights, dimensions, and other parameters.
*
* @access public
* @param mixed $package
@return void
*/
public function calculate_shipping( $package = array() ) {
// Get Country
$country = $package["destination"]["country"];
// Get Postcode
$postcode = $package["destination"]["postcode"];
// Get City
$city = $package["destination"]["city"];
// We're going to use only state field to show shipping methods.
// You can use any according to your needs.
// Selected State from Checkout Fields
$state = $package["destination"]["state"];
$rates = array();
// Shipping Methods will be only shown if State is selected.
if(!empty($state)) {
// Creating Rates/Methods according to State Value
switch($state) {
case 'B':
$rate_1 = array(
'id' => 'bucharest_beefast',
'label' => 'Livrare Rapidă',
'cost' => isset( $this->settings['bucharest_beefast'] ) ? $this->settings['bucharest_beefast'] : 0
);
$rate_2 = array(
'id' => 'bucharest_fan_courier',
'label' => 'Livrare Fan Courier',
'cost' => isset( $this->settings['bucharest_fan_courier'] ) ? $this->settings['bucharest_fan_courier'] : 0
);
array_push($rates, $rate_1);
array_push($rates, $rate_2);
break;
case 'IF':
$rate_1 = array(
'id' => 'ilfov_fan_courier',
'label' => 'Livrare Fan Courier',
'cost' => isset( $this->settings['ilfov_fan_courier'] ) ? $this->settings['ilfov_fan_courier'] : 0
);
array_push($rates, $rate_1);
break;
default:
$rate_1 = array(
'id' => 'gls',
'label' => 'Livrare GLS',
'cost' => isset( $this->settings['gls'] ) ? $this->settings['gls'] : 0
);
array_push($rates, $rate_1);
break;
}
// Adding Rates/Methods to show on Checkout Screen
foreach($rates as $rate) {
$this->add_rate( $rate );
}
}
}
}
}
}
function add_custom_shipping_method( $methods ) {
$methods[] = 'Custom_Shipping_Method';
return $methods;
}
add_filter( 'woocommerce_shipping_methods', 'add_custom_shipping_method' );
}
- That’s It. Go to Plugin in wp-admin dashboard and Activate the plugin.
- Find plugin settings in Woocommerce >> Settings >> Shipping >> Custom Shipping.
- Test Custom Shipping Methods by Adding Products. (Remember to change country code in plugin on line no. 50 or remove it if you’re going to use it for all countries).
- You can modify the code according to your needs.
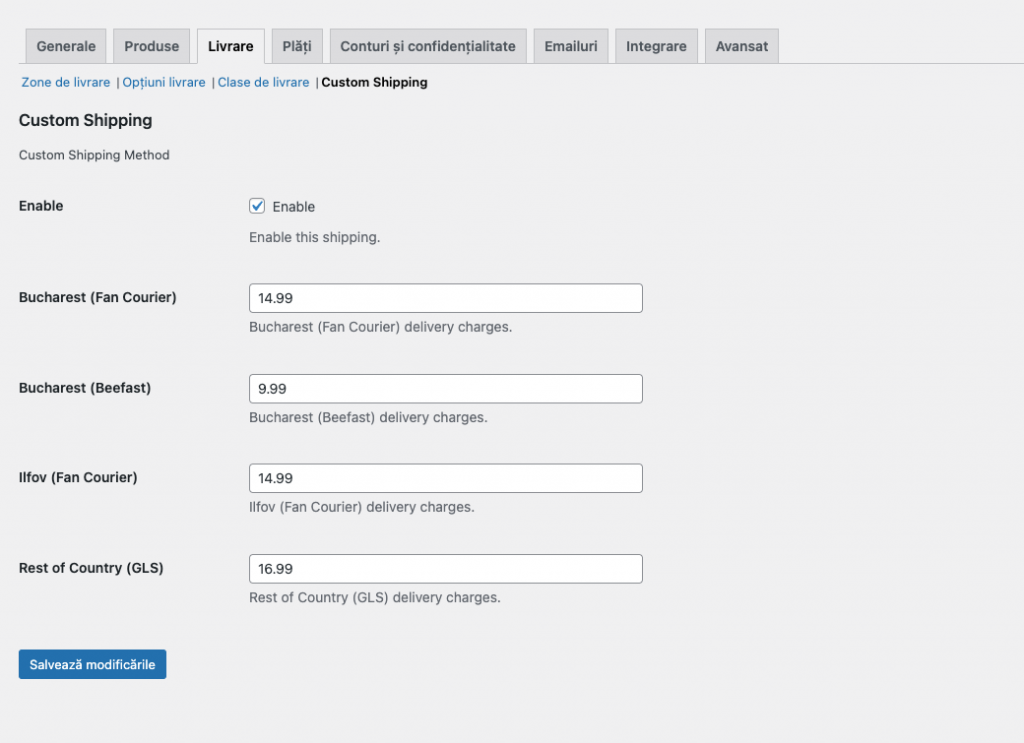
Creating Custom Shipping Methods for Woocommerce is super easy. Still, If you are stuck anywhere feel free to comment on the post, I will reply back with the solution ASAP.